Together, Codebase and AlpineJS can handle many basic animations – several examples are below.
Codebase animation components are about adding and removing Codebase CSS utility classes in response to a trigger event (click, hover, or scroll). Utilities such as translate-*
(i.e. move), rotate-*
, scale-*
, and opacity-*
(for fading) are often used for CSS based animations. However, numeric utilities such as for dimensions, margins, paddings, and colors can also be animated.
Codebase is not a full animation library, and neither is Alpine. If you want to develop a richly animated interactive website, there are several frameworks and platforms out there that you can use. Some require payment, while some are free. Here are a few examples:
- GreenSock Animation Platform (GSAP) – a JavaScript toolset for SVG, WebGL, and Canvas animations
- Anime.js – a JavaScript animation engine
- Animate on Scroll (AOS) – a JavaScript/CSS library for triggering animations when you scroll down to them
Also, “no code” website builders (such as Webflow and Wix) have some element animation effects built in.
Note: with the new scroll-driven animations coming along, many of the animations that designers use these libraries for will have a browser-native way of achieving much the same thing. However, as of November 2024, only Chrome, Edge and other CHromium based browsers can do native scroll-driven animations (not Firefox and Safari). See canIuse: animation-timeline.
Codebase animations can be used in components such as collapse, dropdowns, modals, and offcanvas panels. They can also be used for adding visual interest to block elements on a homepage or landing page.
Animation trigger events
Here are two options:
- On click (or on tap, for touch-screen devices). “On click” triggered animations can be tapped repeatedly and they will be triggered every time.
- On scroll into view (using the Alpine Intersect plugin). “On scroll” animations can be set to trigger once, when the visitor scrolls down to them the first time, or they can be set to trigger repeatedly whenever the visitor scrolls down or up through them. I.e. When the visitor scrolls beyond this animation, it can be reset so that it is ready to be triggered again.
On click (on tap)
<div x-data="{ activated: false }">
<div
@click="activated = !activated"
tabindex="0"
@keydown.enter="activated = !activated"
aria-controls="panel-1"
:aria-expanded="activated"
@keyup.escape="activated = false"
id="panel-1"
class="w-50% p-2 bg-green-200 transition-transform-600ms"
:class="activated && 'translate-right-100%'"
>
<strong>Click me, and I will move.</strong> Click me again, and I will return to my original position.
</div>
</div>
On hover (a.k.a. mouseover) is not recommended for animations
While it is also possible to animate on hover (a.k.a. on mouseover), it is not recommended in most situations because you also have to support touch screens. “On hover” behaves as a tap plus focus for touch screen devices, and tapping outside the “on hover” element will leave the focus on that element. This means that you would have to tap away a second time (or double tap) in order to remove the focus. But that will confuse your visitors.
Instead, use “on click” because it is more intuitive. It behaves as your visitor would expect it to – and provides essentially the same experience whether using a touch screen or a pointing device (mouse or other).
(Hovever, “on hover” can sometimes be used in situations where there is little or no animations, and where leaving the focus behind may not matter so much – e.g. on dropdown menus and tooltips.)
On scroll into view
The scroll animation above occurs once. To see it happen again, refresh your browser.
<div
x-data="{ activated: false }"
x-intersect:enter="activated = true"
>
<div
id="panel-2"
class="w-50% p-2 bg-green-200 transition-transform-600ms"
x-show="activated"
x-transition:enter="translate-right-100%"
>
When you scroll down to me, I move.
</div>
</div>
Animation timing
Besides the three trigger events documented above, Cosebase also has seversl CSS built-in transition
timings, that you can use to control faster or slower animations. Animation speeds are controlled in milliseconds (ms).
- Transition all:
transition-all-150ms
/transition-all-300ms
/transition-all-600ms
/transition-all-900ms
/transition-all-1200ms
/transition-all-1500ms
/transition-all-1800ms
/transition-all-2400ms
- Transition CSS transforms only:
transition-transform-150ms
/transition-transform-300ms
/transition-transform-600ms
/transition-transform-900ms
/transition-transform-1200ms
/transition-transform-1500ms
/transition-transform-1800ms
/transition-transform-2400ms
You’ve seen transition-transform-600ms
being used in the demo examples above.
Animation utilities
Translate (move)
translate-up-100%
/translate-right-100%
/translate-down-100%
/translate-left-100%
/translate-0
Example: translate-right-100%
(with timing transition-transform-600ms
)
A translation animation is also used in the offcanvas component. The offcanvas-panel
classes merely position the panel in is expanded (revealed) location. Use one of the directional translation classes to start it hidden off canvas, and then use translate-0
to reveal it (translate-0
works for both the x and y axis) .
Grow and shrink
Scales both in the X and Y axis, retaining the elements proportions.
scale-0
/scale-25%
/scale-50%
/scale-75%
/scale-100%
/scale-125%
/scale-150%
/scale-175%
/scale-200%
scale-*
utilities scale from the center-middle of an element. If you want to grow from or shrink to an edge or corner, add in a transform-origin-*
utility.
Example: scale-150%
A scale animation has also been added to a dropdown demo and a modal demo.
Rotate
rotate-90
/rotate-minus90
/rotate-180
/rotate-minus180
/rotate-270
rotate-*
utilities pivot aroung the center-middle of an element. If you want to pivot around a corner or center/middle of an edge, add in a transform-origin-*
utility.
Example: rotate-180
A rotation is also used in a collapse demo example for rotating a caret icon. A similar example us used in the sidebar menu of these docs.
Transform origin
The transform origin of elements defalts to its center-middle. You can change that by adding one of the following transform-origin-*
utilities:
- (Center or middle of) edges:
transform-origin-top
/transform-origin-right
/transform-origin-bottom
/transform-origin-left
/ - Corners:
transform-origin-top-right
/transform-origin-bottom-right
/transform-origin-bottom-left
.transform-origin-top-left
Example: transform-origin-top
has been used on the dropdown animation demo.
Opacity
opacity-0
/opacity-25%
/opacity-50%
/opacity-75%
/opacity-100%
hover:opacity-0
/hover:opacity-25%
/hover:opacity-50%
/hover:opacity-75%
/hover:opacity-100%
Example fading to opacity-50%
:
Notes on animating opacity
- Unlike the other examples above, in CSS
opacity
is not a CSS transform. Therefore you need to usetransition-all-*
(nottransition-transform-*
). - There is also another way to animate opacity: you can pair the opacity utilities with
fade-in
orfade-out
(see fade-in/out below). - AlpineJS has its own (JavaScript powered) directive modifier,opacity on x-transition, that you can use on an
x-show
.
Flip
flip-*
utilities can be used to “turn over” cards or other block element, to show their back face:
flip-x
– flips the element vertically (the pivot is on the x-axis)flip-y
- flips the element horizontally (the pivot is on the y-axis)
Ordinarily, the back side of an element is a “mirror image” of the front face. If that’s not what you want a visitor to see, add the CSS utility backface-hidden
. Then the back face will simply be transparent (so, the wrapping element background or page background will be seen instead).
In some circumatances, you may also need to add the CSS utility preserve-3d
to the wrapper of the flipped element.
Example: flip-y
Example: flip-y backface-hidden
You can’t click a flipped backface-hidden
element. So, to make the example above reappear, you will need to refresh your browser.
Fade-in/out and scale-in/out
In addition to the above, a few simple keyframes animations have been provied. These are not for use in AlpineJS x-transition
but they can be used in x-bind:class
that is triggered by a change of state. These all have built-in animation
timings of 300ms.
fade-in
– goes from 0 to 1 (100%) opacity (transparent to fully visible)fade-out
– goes from 1 to 0 opacity (fully viible to transparent).scale-in
– goes from 75% to 100% transform scalescale-out
– goes from 100% to 75% transform scale
These can be useful for revealing and hiding panels.
Fade-in/out
Example fading. Here a ternary class toggler is required in the Alpine component:
:class="activated ? 'fade-out opacity-0' : 'fade-in opacity-100%'"
The The faded out (invisible) element is still present, therefore it can be clicked. So, click inside the dashed area above and the invisible element will fade in again.
Scale-in/out
Example fading. Here a ternary class toggler is required in the Alpine component:
:class="activated ? 'scale-out scale-75%' : 'scale-in scale-100%'"
scale-in
has also been used on the example embedded popout modal.
Other CSS utilities that can be animated
You can animate any style that has a numeric setting. So, you can animate using Codebase utility classes for dimensions, spacing (margins, paddings), border (thickness), and colors.
Note: These other syles are CSS transforms. Therefore you need to use transition-all-*
(not transition-transform-*
).
Example color animation:
:class="activated ? 't-black bg-white' : 't-white bg-black'"
Examples
Featured image and text card
With two components (one in each grid cell) that are triggered to true
when they enter the viewport on scroll, using x-intersect
both on entering and leaving the viewport. So, these animations will be triggered every time the panels are scrolled into the viewport, whether you scroll down or up.
Lorem, ipsum dolor sit amet consectetur adipisicing elit. Quo facere velit tempora fugiat nulla blanditiis.
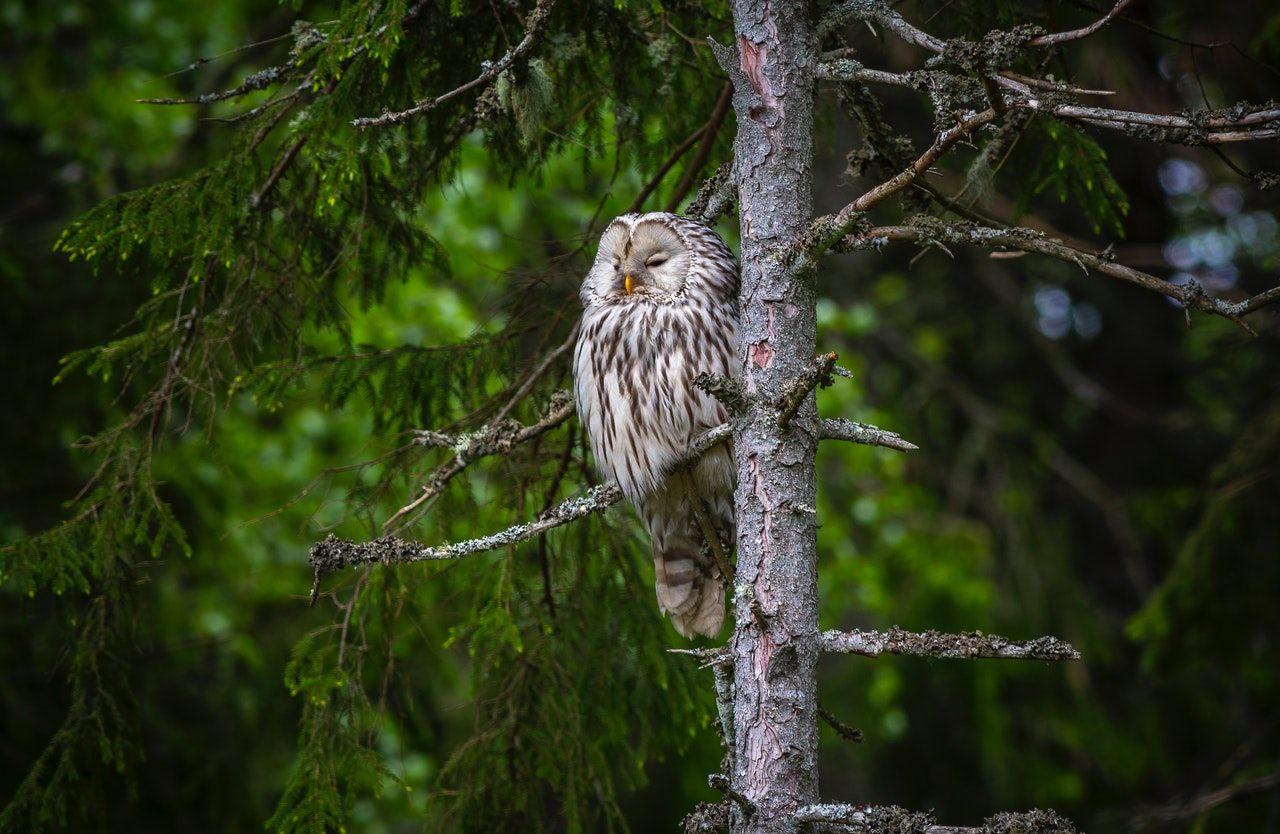
Team reveal
Click/tap to activate. (This could just as easily have been set to activate on scroll, using x-intersect
.)
Sliding panels
Two panels that fade in and slide together from right and left, on scroll.
For this example, these will be triggered every time the panels are scrolled into the viewport, whether you scroll down or up.